python open找不到文件
在使用Python进行文件操作时,经常会遇到`open`函数找不到文件的情况。这种情况可能由于多种原因引起,比如文件路径错误、文件不存在、权限问题等。本文将详细探讨这些问题,并提供相应的解决方案。
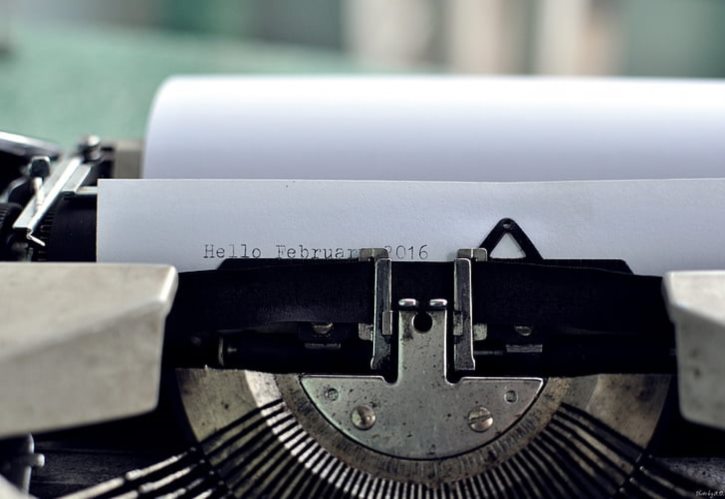
1. 文件路径错误
最常见的原因之一是文件路径错误。在Python中,`open`函数需要一个准确的路径来找到文件。如果路径不正确,Python将无法找到文件并会抛出`FileNotFoundError`异常。
示例代码:
```python
try:
with open('wrong_file.txt', 'r') as file:
content = file.read()
except FileNotFoundError as e:
print(f"Error: {e}")
```
解决方案:
确保提供的路径是正确的。可以使用绝对路径或相对路径,但必须确保路径中的每个目录都存在。可以通过以下方式检查路径:
```python
import os
file_path = 'correct_file.txt'
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
else:
print("File does not exist.")
```
2. 文件不存在
如果指定的文件根本不存在,Python也会抛出`FileNotFoundError`异常。这种情况通常发生在尝试打开一个新创建的文件或在错误的目录中查找文件时。
示例代码:
```python
try:
with open('nonexistent_file.txt', 'r') as file:
content = file.read()
except FileNotFoundError as e:
print(f"Error: {e}")
```
解决方案:
在打开文件之前,先检查文件是否存在。可以使用`os.path.exists`函数来验证文件的存在性:
```python
import os
file_path = 'existing_file.txt'
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read() else: print("File does not exist.") ```
3. 权限问题有时,即使文件路径正确且文件存在,也可能因为权限问题而无法打开文件。例如,尝试读取一个只读文件或写入一个只读文件时,Python会抛出`PermissionError`异常。 示例代码: ```python try: with open('readonly_file.txt', 'w') as file: file.write('This is a test.') except PermissionError as e: print(f"Error: {e}") ``` 解决方案:确保有足够的权限来访问或修改文件。可以通过更改文件权限或以管理员身份运行脚本来解决这个问题。 ```python import os os.chmod('writable_file.txt', 0o777) 更改权限为可读写执行 try: with open('writable_file.txt', 'w') as file: file.write('This is a test.') except PermissionError as e: print(f"Error: {e}") ``` 4. UnicodeDecodeError在处理文本文件时,可能会遇到编码问题导致的`UnicodeDecodeError`异常。这通常发生在尝试以错误的编码格式读取文件时。 示例代码: ```python try: with open('encoded_file.txt', 'r', encoding='utf-8') as file: content = file.read() except UnicodeDecodeError as e: print(f"Error: {e}") ``` 解决方案:确保使用正确的编码格式打开文件。可以通过指定正确的编码参数来解决这个问题: ```python try: with open('encoded_file.txt', 'r', encoding='utf-8') as file: content = file.read() except UnicodeDecodeError as e: print(f"Error decoding the file using UTF-8 encoding.") ``` 总结通过以上章节的详细讲解,我们了解了在使用Python的`open`函数时可能遇到的多种问题及其解决方案。无论是路径错误、文件不存在、权限问题还是编码问题,都可以通过适当的检查和调整来避免这些错误的发生。
本站所有图文均由用户自行上传分享,仅供网友学习交流。若您的权利被侵害,请联系 KF@Kangenda.com